Documentation
API
Our API documentation provides a comprehensive guide to integrating and using our platform’s features efficiently.
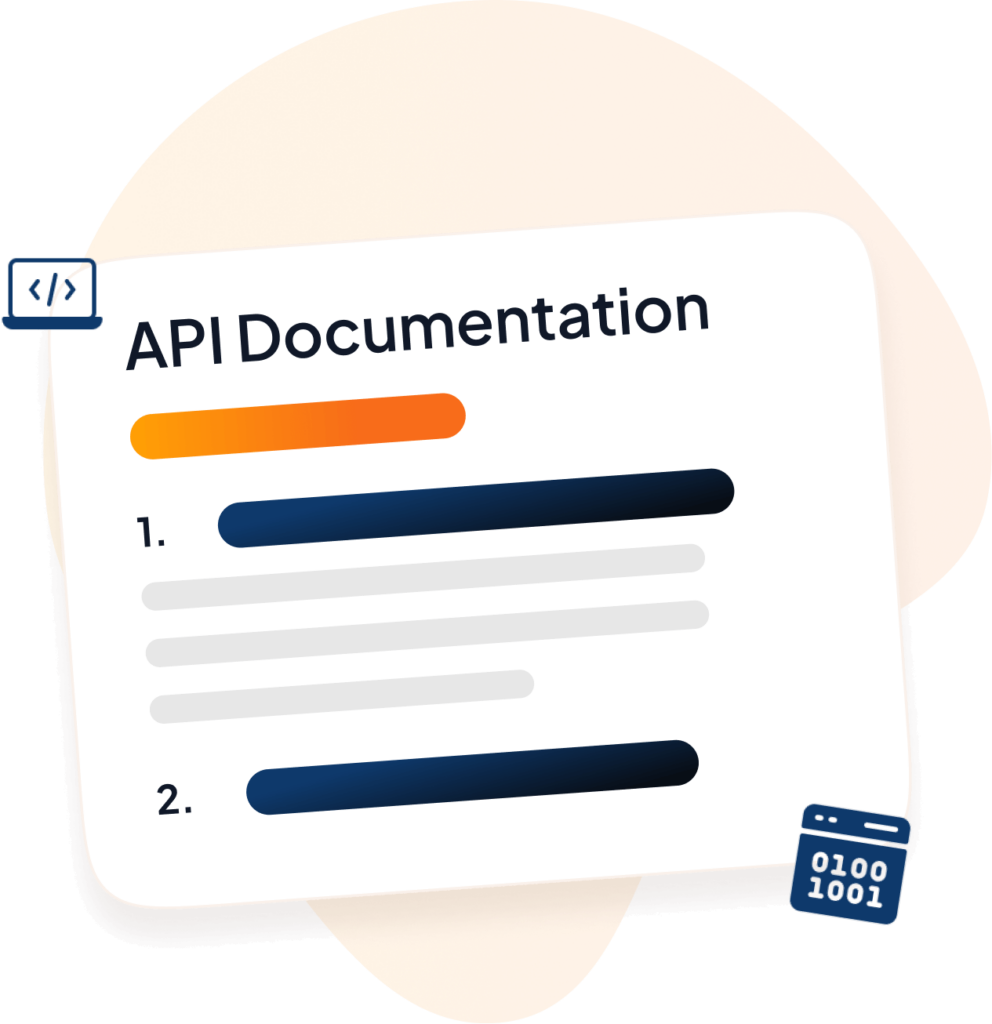
Unduit APIS
Welcome to the Unduit API Collection
The Unduit API collection enables developers to seamlessly integrate their platforms with the Unduit system. Using these APIs, you can access and interact with data related to your company on the Unduit platform.
Purpose
This collection provides endpoints to:
- Authenticate and manage access securely using tokens.
- Fetch and manage records relevant to your company.
- Support seamless integrations with high efficiency and security.
Key Features
- Secure Access: Every request requires proper authentication, ensuring secure data handling.
- Company-Specific Data: All APIs are scoped to provide data that is specific to your company in the Unduit system.
- Ease of Use: Example requests and detailed documentation included for each endpoint.
- Scalable Integration: Built to handle multiple integrations and high-volume requests efficiently.
- Standardized Error Handling: Clear error codes are provided for better debugging and integration management.
Error Codes
The Unduit API follows standard HTTP status codes for responses:
Code | Description |
---|---|
200 | Successful response. |
400 | Bad request (e.g., missing parameters). |
401 | Unauthorized (e.g., invalid or expired token). |
404 | Not found (e.g., invalid endpoint or resource). |
422 | Validation error (e.g., invalid input format). |
500 | Server error (e.g., an unexpected issue). |
Getting Started
- Import this Postman collection into your workspace.
- Set up an environment with the following variables:
base_url
:https://dev-api.unduit.com/api/v1
auth_token
: The Bearer Token obtained from the Login API.
- Follow the detailed instructions provided in each API folder to understand specific functionality.
Support
If you have any questions or need assistance, feel free to reach out to the Unduit team at [email protected].
Authentication
The Authentication folder contains endpoints required to securely access the Unduit API. These APIs help you manage your access tokens and ensure secure interactions with the platform.
Endpoints in this Folder
- Login API: Obtain an access token by authenticating with your email and password.
Refresh Token API: Renew your access token when it is about to expire.
1. Login API
Purpose:
Authenticate a user and retrieve a JSON Web Token for subsequent API requests. This token is required to authorize every request to the Unduit APIs.
Endpoint:
POST https://dev-api.unduit.com/api/v1/login
Request Structure
{ "email": "your-email@example.com", "password": "your-password"}
Response
On successful login, you’ll receive a token valid for 4 hours.
Example Response:
{
"message": "Login successful",
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MiwiZW1haWwiOiJmYWlyd2F5aW5kZXBlbmRlbnRtb3J0Z2FnZWNvcnBvcmF0aW9uQHVuZHVpdC5jb20iLCJjb21wYW55X2lkIjozMTcsImlhdCI6MTczMzMxNTY4OCwiZXhwIjoxNzMzMzE5Mjg4fQ.RNon3WMwoBrz0C0HZxi3TDSt-LjIwL38Wnrue6yvCVw"
}
Error Handling
If the credentials are invalid, you’ll receive an error response:
{
"message": "Invalid email or password"
}
2. Refresh Token API
If the credentials are invalid, you’ll receive an error response:
Purpose:
Renew an expired or soon-to-expire token to maintain uninterrupted access to the API.
Endpoint:
POST https://dev-api.unduit.com/api/v1/refresh-token
Request Structure:
- Body: (Raw JSON)
Example Request
{
"refresh_token": "your_current_token"
}
Response
On success, you’ll receive a new token valid for the next 4 hours.
Example Response:
{
"message": "Token refreshed successfully",
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MiwiZW1haWwiOiJmYWlyd2F5aW5kZXBlbmRlbnRtb3J0Z2FnZWNvcnBvcmF0aW9uQHVuZHVpdC5jb20iLCJjb21wYW55X2lkIjozMTcsImlhdCI6MTczMzIxNTc4OCwiZXhwIjoxNzMzMzE5Mjg4fQ.RNon3WMwoBrz0C0HZxi3TDSt-LjIwL38Wnrue6yvCVw"
}
Error Handling:
If the token is invalid or expired, you’ll receive an error response
{
"message": "Invalid token"
}
Usage Notes
- Always include the Bearer Token in the Authorization header for secured endpoints.
- Tokens expire after 4 hours. Use the Refresh Token API to renew them before expiry.
- Ensure secure storage of tokens on the client-side to prevent unauthorized access.
POST Login
https://dev-api.unduit.com/api/v1/auth/login
Example Request
curl --location 'https://dev-api.unduit.com/api/v1/auth/login' \
--data-raw '{
"email": "jane.smith@example.com",
"password": "password"
}'
Example Response
{
"message": "Login successful",
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MiwiZW1haWwiOiJmYWlyd2F5aW5kZXBlbmRlbnRtb3J0Z2FnZWNvcnBvcmF0aW9uQHVuZHVpdC5jb20iLCJjb21wYW55X2lkIjozMTcsImlhdCI6MTczMzM5Mzk5NSwiZXhwIjoxNzMzMzk3NTk1fQ.qvWAgXO-AUr5-JurBC_cBMJ1y-SWJmkcTlihS-n5gjw"
}
POST Refresh Token
https://dev-api.unduit.com/api/v1/refresh-token
Example Request
curl --location 'https://dev-api.unduit.com/api/v1/refresh-token' \
--data '{
"refresh_token": ""
}'
Example Request
{
"message": "Token has been refreshed successfully.",
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MiwiZW1haWwiOiJmYWlyd2F5aW5kZXBlbmRlbnRtb3J0Z2FnZWNvcnBvcmF0aW9uQHVuZHVpdC5jb20iLCJjb21wYW55X2lkIjozMTcsImlhdCI6MTczMzM5Mzk5NSwiZXhwIjoxNzMzMzk3NTk1fQ.qvWAgXO-AUr5-JurBC_cBMJ1y-SWJmkcTlihS-n5gjw"
}
Manage
The Manage folder provides APIs to manage users and assets associated with a company on the Unduit platform. These APIs enable developers to retrieve lists of users and assets, view detailed information, and filter assets based on specific criteria. Below is a detailed breakdown of the APIs within this folder:
API Endpoints
- Endpoint:
https://dev-api.unduit.com/api/v1/users
- Description: Retrieves a paginated list of users associated with the company. Each page contains up to 10 records by default. Developers can apply filters, such as by email, to narrow down the results. Each user object includes an
AssignedAssets
array, which contains all assets assigned to the user. If no asset is assigned, this array will be empty.
Example Request:
Method: GET
Get all users (default behaviour)https://dev-api.unduit.com/api/v1/users?page=1
- Retrieves the first page of users, containing up to 10 records per page.
- Filter by email:
https://dev-api.unduit.com/api/v1/users?page=1&[email protected]
- Retrieves users matching the provided email address.
Query Parameters:
Parameter | Type | Description |
---|---|---|
page | Number | Specifies the page number for pagination. Default is 1. |
String | (Optional) Filters users by email address. Returns matching users only. |
Example Response
{
"totalUsers": 465,
"totalPages": 47,
"currentPage": 1,
"users": [
{
"FirstName": "John",
"LastName": "Doe",
"LocationName": null,
"PhoneCountryCode": "US",
"ContactInfo": "1234567890",
"Email": "john.doe@example.com",
"Country": "US",
"State": "CA",
"City": "Los Angeles",
"Zip": "90001",
"LocationType": "Remote Employee",
"Address_1": "1234 Elm Street",
"Address_2": "",
"AssignedAssets": []
},
{
"FirstName": "Jane",
"LastName": "Smith",
"LocationName": null,
"PhoneCountryCode": "US",
"ContactInfo": "0987654321",
"Email": "jane.smith@example.com",
"Country": "US",
"State": "NY",
"City": "New York",
"Zip": "10001",
"LocationType": "Remote Employee",
"Address_1": "5678 Oak Avenue",
"Address_2": "Unit 202",
"AssignedAssets": [
{
"assetId": "LEN-0000-ABCD1234",
"modelName": "Demo Laptop",
"modelNumber": "DL-1234",
"productType": "Laptop",
"purchaseDate": "2024-10-31",
"serialNumber": "SN-ABCD1234",
"imei": "",
"imei2": "",
"sku": "",
"assetStatus": "In Use",
}
]
}
]
}
2. Asset List
Method: GET
Endpoint: https://dev-api-v1.unduit.com/api/v1/assets
Description: Retrieves a paginated list of all assets associated with the company. Each page contains up to 10 records by default. Developers can apply filters such as serialNumber and assetId to narrow down the results. Each asset object includes an AssignedUser object, which contains the name and email of the user to whom the device is assigned. If the device is unassigned, the AssignedUser object will be null.
Method: GET
- Get all assets (default behaviour):
-
https://dev-api-v1.unduit.com/api/v1/assets?page=1
- Retrieves the first page of assets, containing up to 10 records per page.
-
- Filter by serial number:
-
https://dev-api-v1.unduit.com/api/v1/assets?page=1&serialNumber=MBP2022XYZ
- Retrieves assets with the specified serial number.
-
- Filter by asset ID:
-
https://dev-api-v1.unduit.com/api/v1/assets?page=1&assetId=101
- Retrieves assets with the specified asset ID.
-
- Filter by both serial number and asset ID:
-
https://dev-api-v1.unduit.com/api/v1/assets?page=1&serialNumber=MBP2022XYZ&assetId=101
- Retrieves assets that match both the specified serial number and asset ID.
-
Query Parameters:
Parameter | Type | Description |
---|---|---|
page | Number | Specifies the page number for pagination. Default is 1. |
serialNumber | String | (Optional) Filters assets by serial number. |
assetId | String | (Optional) Filters assets by ID. |
Example Response
{
"currentPage": 1,
"totalPages": 53,
"totalItems": 523,
"assets": [
{
"assetId": "DEMO-ASSET-123",
"modelName": "Demo Device",
"modelNumber": "Model X",
"productType": "Laptop",
"purchaseDate": "2024-01-01",
"serialNumber": "SN123456",
"imei": "",
"imei2": "",
"sku": "SKU123",
"assetStatus": "In Use",
"AssignedUser": {
"firstname": "John",
"lastname": "Doe",
"username": "John Doe",
"email": "john.doe@example.com"
}
},
{
"assetId": "DEMO-ASSET-456",
"modelName": "Demo Device",
"modelNumber": "Model Y",
"productType": "Tablet",
"purchaseDate": "2024-02-01",
"serialNumber": "SN654321",
"imei": "",
"imei2": "",
"sku": "SKU456",
"assetStatus": "In Use",
"AssignedUser": {
"firstname": "Jane",
"lastname": "Smith",
"username": "Jane Smith",
"email": "jane.smith@example.com"
}
}
]
}
Key Notes
- These APIs allow developers to efficiently manage user and asset data for their company on the Unduit platform.
- Be mindful of user and asset identifiers when making requests to ensure accurate results.
- Always secure your API calls with a valid token as described in the Authentication section.
GET Users List
Example Request
curl --location 'https://dev-api.unduit.com/api/v1/users?page=1' \
--header 'Authorization: Bearer '
Example Response
{
"totalUsers": 465,
"totalPages": 47,
"currentPage": 1,
"users": [
{
"FirstName": "John",
"LastName": "Doe",
"LocationName": null,
"PhoneCountryCode": "US",
"ContactInfo": "1234567890",
"Email": "john.doe@example.com",
"Country": "US",
"State": "CA",
"City": "Los Angeles",
"Zip": "90001",
"LocationType": "Remote Employee",
"Address_1": "1234 Elm Street",
"Address_2": "",
"AssignedAssets": []
},
{
"FirstName": "Jane",
"LastName": "Smith",
"LocationName": null,
"PhoneCountryCode": "US",
"ContactInfo": "0987654321",
"Email": "jane.smith@example.com",
"Country": "US",
"State": "NY",
"City": "New York",
"Zip": "10001",
"LocationType": "Remote Employee",
"Address_1": "5678 Oak Avenue",
"Address_2": "Unit 202",
"AssignedAssets": [
{
"assetId": "LEN-0000-ABCD1234",
"modelName": "Demo Laptop",
"modelNumber": "DL-1234",
"productType": "Laptop",
"purchaseDate": "2024-10-31",
"serialNumber": "SN-ABCD1234",
"imei": "",
"imei2": "",
"sku": "",
"assetStatus": "In Use",
}
]
}
]
}
GETAssets List
Example Request
curl --location 'https://dev-api.unduit.com/api/v1/assets?page=1' \
--header 'Authorization: Bearer '
Example Response
{
"currentPage": 1,
"totalPages": 53,
"totalItems": 523,
"assets": [
{
"assetId": "DEMO-ASSET-123",
"modelName": "Demo Device",
"modelNumber": "Model X",
"productType": "Laptop",
"purchaseDate": "2024-01-01",
"serialNumber": "SN123456",
"imei": "",
"imei2": "",
"sku": "SKU123",
"assetStatus": "In Use",
"AssignedUser": {
"firstname": "John",
"lastname": "Doe",
"username": "John Doe",
"email": "john.doe@example.com"
}
},
{
"assetId": "DEMO-ASSET-456",
"modelName": "Demo Device",
"modelNumber": "Model Y",
"productType": "Tablet",
"purchaseDate": "2024-02-01",
"serialNumber": "SN654321",
"imei": "",
"imei2": "",
"sku": "SKU456",
"assetStatus": "In Use",
"AssignedUser": {
"firstname": "Jane",
"lastname": "Smith",
"username": "Jane Smith",
"email": "jane.smith@example.com"
}
}
]
}